Make sitemap.xml markdown blog | A script to create sitemap.xml
Tue Jan 05 2021
- D. K. Dhabale
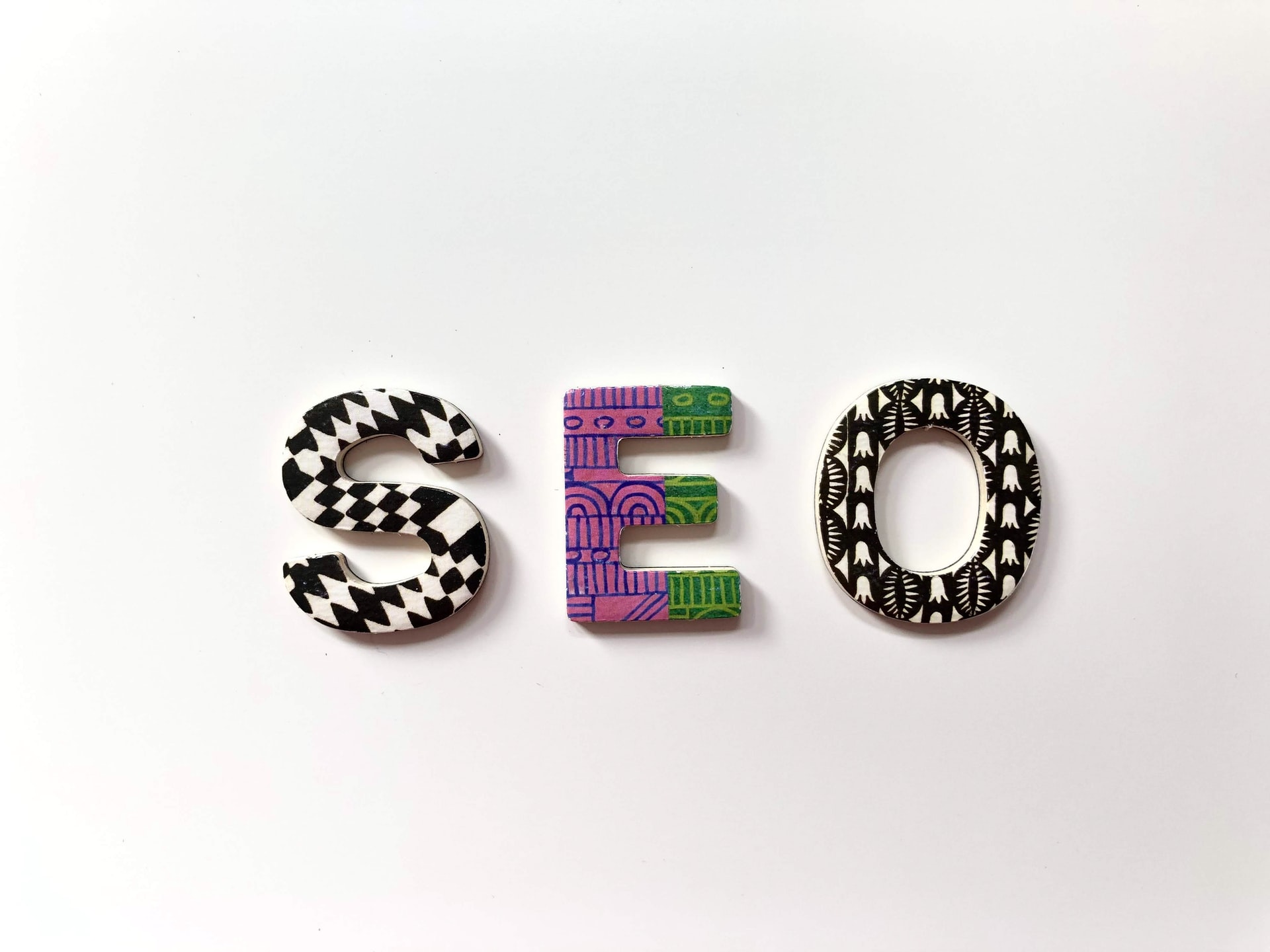
Script buildTools/buildSitemap.mjs
Read the comments and place your data where required.
Dependencies - fs, path, gray-matter
import fs from "fs"import { join } from "path"import matter from "gray-matter";(() => {// NOTE: Replace with your site's urlconst site = "https://darshandev.tech/"// NOTE: The directory where you store your posts, w.r.t. project rootconst data = fs.readdirSync("./src/mdx/posts")// NOTE: The posts directory, but without ./ in the beginningconst postsDirectory = join(process.cwd(), "src/mdx/posts")console.log(data)const siteMapData = data.map((slug) => {//NOTE: Use the extension that you use in your blogs, md or mdxconst realSlug = slug.replace(/\.mdx$/, "")const fullPath = join(postsDirectory, `${realSlug}.mdx`)const fileContents = fs.readFileSync(fullPath, "utf8")const { data } = matter(fileContents)return { url: site + realSlug, date: data.date }})const sitemap = `<?xml version="1.0" encoding="UTF-8"?><urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">${siteMapData.map((blog) => {return `<url><loc>${blog.url}</loc><lastmod>${new Date(blog.date).toISOString()}</lastmod><changefreq>monthly</changefreq><priority>1.0</priority></url>`}).join("")}</urlset>`// NOTE: The output locationfs.writeFileSync("./public/sitemap.xml", sitemap)})()
Adding script to package.json
Add this script to package.json scripts object,
"prebuild":"node ./src/buildTools/buildSitemap.mjs",
This will run your script before running build.
Bonus: SEO Tip
You can submit your sitemap to the Google search console and request google to index it. Google Search Console
References
NPM Scripts docs - https://docs.npmjs.com/cli/v8/using-npm/scripts