useEffect: A quick guide to the react hook
Sat Jan 01 2022
- D. K. Dhabale
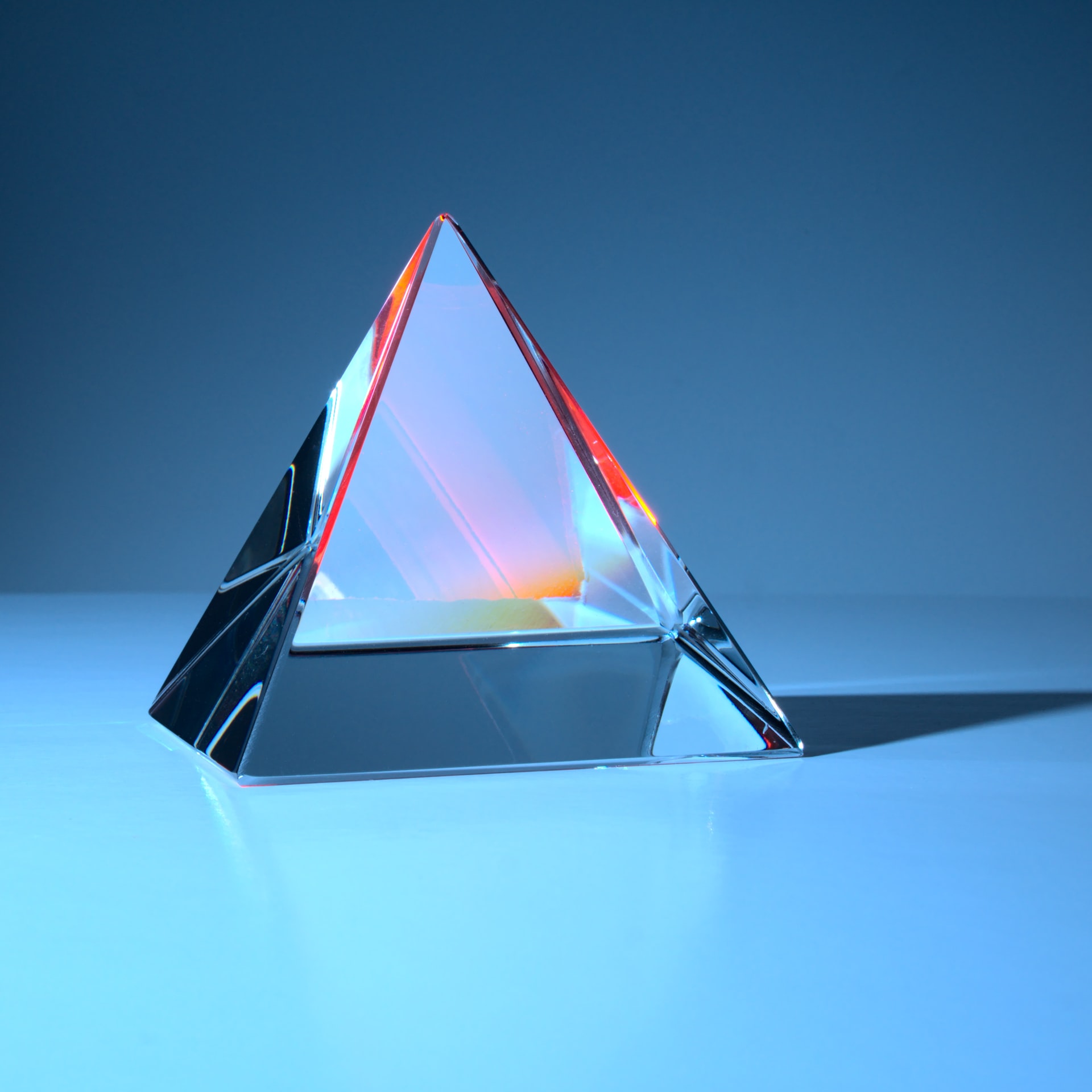
Lets learn useEffect
You must know how to use functional components and the useState hook
useEffect is a very simple hook. It lets you run a piece of code when a certain variable changes.
import { useEffect } from "react"export default function MyComponent(){useEffect(()=>{console.log("component rendered");}, []);return(<p>This is a component</p>);}
Params
useEffect( func, dependencyList )
func: This is the function to run when a variable in the dependencyList changes.
dependencyList: This is the list of variables that trigger the fucntion above to fire.
The dependencyList can be empty, which will cause the function to trigger just once, i.e. when the component mounts.
Cleanup function
You can return a function from func, this function will be executed when the component unmounts or
a variable in the dependencyList changes.
If the dependencyList is empty, the cleanup fucntion will only run when the component unmounts (gets removed from DOM).
Example of the cleanup fucntion:
import { useEffect } from "react"export default function MyComponent(){useEffect(()=>{console.log("component rendered");return(()=>{console.log("Cleanup!");console.log("You can use your unsubscribe methods here, and remove any event listeners that you no longer need.");})}, []);return(<p>This is a component</p>);}